3. General Examples#
A place to keep processing or other functionality that may lie outside of the standard pipeline.
import lbm_suite2p_python.volume
%load_ext autoreload
%autoreload 2
from pathlib import Path
import os
import matplotlib.pyplot as plt
import numpy as np
import suite2p
import mbo_utilities as mbo
from copy import deepcopy
import lbm_suite2p_python as lsp
import fastplotlib as fpl
import tifffile
import matplotlib as mpl
mpl.rcParams.update({
'axes.spines.left': True,
'axes.spines.bottom': True,
'axes.spines.top': False,
'axes.spines.right': False,
'legend.frameon': False,
'figure.subplot.wspace': .01,
'figure.subplot.hspace': .01,
'figure.figsize': (18, 13),
'ytick.major.left': True,
})
jet = mpl.cm.get_cmap('jet')
jet.set_bad(color='k')
The autoreload extension is already loaded. To reload it, use:
%reload_ext autoreload
3.1. Assembly#
To preview data, run
scan = mbo.read_scan(files, join_contiguous=True)
widget = mbo.run_gui(scan)
widget.show()
scan = mbo.read_scan(r"D:\W2_DATA\kbarber\2025-02-27\mk301\green\*", join_contiguous=True)
animal_path = Path(r"D:\W2_DATA\kbarber\2025-02-27\mk301")
assembled_path = animal_path.joinpath("assembled")
save_path = animal_path.joinpath("results")
print(f"Saving raw tiffs to: {assembled_path}")
print(f"Saving suite2p results to: {save_path}")
Saving raw tiffs to: D:\W2_DATA\kbarber\2025-02-27\mk301\assembled
Saving suite2p results to: D:\W2_DATA\kbarber\2025-02-27\mk301\results
input_files = mbo.get_files(assembled_path, str_contains='tif', max_depth=3)
input_files = [Path(x) for x in input_files]
input_files[:3]
[WindowsPath('D:/W2_DATA/kbarber/2025-02-27/mk301/assembled/plane_01.tiff'),
WindowsPath('D:/W2_DATA/kbarber/2025-02-27/mk301/assembled/plane_02.tiff'),
WindowsPath('D:/W2_DATA/kbarber/2025-02-27/mk301/assembled/plane_03.tiff')]
ops_files = mbo.get_files(save_path.parent, 'ops', 4)
ops_files[:3]
['D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\assembled\\suite2p\\plane0\\ops.npy',
'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results\\plane_01\\plane0\\ops.npy',
'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results\\plane_02\\plane0\\ops.npy']
ops0 = lsp.load_ops(ops_files[-1])
lsp.plot_segmentation(ops0, './test_seg_overlay.png')
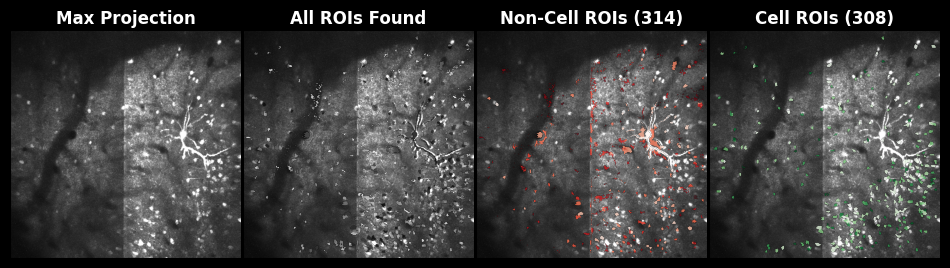
output_ops = ops0
stats_file = Path(output_ops['save_path']).joinpath('stat.npy')
iscell = np.load(Path(output_ops['save_path']).joinpath('iscell.npy'), allow_pickle=True)[:, 0].astype(int)
stats = np.load(stats_file, allow_pickle=True)
print(stats[0].keys())
dict_keys(['ypix', 'xpix', 'lam', 'med', 'footprint', 'mrs', 'mrs0', 'compact', 'solidity', 'npix', 'npix_soma', 'soma_crop', 'overlap', 'radius', 'aspect_ratio', 'npix_norm_no_crop', 'npix_norm', 'skew', 'std', 'neuropil_mask'])
ops0
{'suite2p_version': '0.14.4',
'look_one_level_down': False,
'fast_disk': 'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results',
'delete_bin': False,
'mesoscan': False,
'bruker': False,
'bruker_bidirectional': False,
'h5py': [],
'h5py_key': 'data',
'nwb_file': '',
'nwb_driver': '',
'nwb_series': '',
'save_path0': 'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results',
'save_folder': 'plane_01',
'subfolders': [],
'move_bin': False,
'nplanes': 1,
'nchannels': 1,
'functional_chan': 1,
'tau': 1.5,
'fs': 17.06863078416647,
'force_sktiff': False,
'frames_include': -1,
'multiplane_parallel': False,
'ignore_flyback': [],
'preclassify': 0.0,
'save_mat': False,
'save_NWB': False,
'combined': True,
'aspect': np.float64(1.0),
'do_bidiphase': 0,
'bidiphase': 0,
'bidi_corrected': False,
'do_registration': True,
'two_step_registration': False,
'keep_movie_raw': False,
'nimg_init': 300,
'batch_size': 500,
'maxregshift': 0.1,
'align_by_chan': 1,
'reg_tif': False,
'reg_tif_chan2': False,
'subpixel': 10,
'smooth_sigma_time': 0,
'smooth_sigma': 1.15,
'th_badframes': 1.0,
'norm_frames': True,
'force_refImg': False,
'pad_fft': False,
'nonrigid': True,
'block_size': [128, 128],
'snr_thresh': 1.2,
'maxregshiftNR': 5,
'1Preg': False,
'spatial_hp_reg': 42,
'pre_smooth': 0,
'spatial_taper': 40,
'roidetect': True,
'spikedetect': True,
'sparse_mode': True,
'spatial_scale': 0,
'connected': True,
'nbinned': 5000,
'max_iterations': 20,
'threshold_scaling': 1.0,
'max_overlap': 0.75,
'high_pass': 100,
'spatial_hp_detect': 25,
'denoise': False,
'anatomical_only': 0,
'diameter': 0,
'cellprob_threshold': 0.0,
'flow_threshold': 1.5,
'spatial_hp_cp': 0,
'pretrained_model': 'cyto',
'soma_crop': True,
'neuropil_extract': True,
'inner_neuropil_radius': 2,
'min_neuropil_pixels': 350,
'lam_percentile': 50.0,
'allow_overlap': False,
'use_builtin_classifier': False,
'classifier_path': '',
'chan2_thres': 0.65,
'baseline': 'maximin',
'win_baseline': 60.0,
'sig_baseline': 10.0,
'prctile_baseline': 8.0,
'neucoeff': 0.7,
'dx': 2.0,
'dy': 2.0,
'tiff_list': ['plane_01.tiff'],
'data_path': ['D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\assembled'],
'input_format': 'tif',
'save_path': 'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results\\plane_01\\plane0',
'ops_path': 'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results\\plane_01\\plane0\\ops.npy',
'reg_file': 'D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\results\\suite2p\\plane0\\data.bin',
'first_tiffs': array([ True]),
'frames_per_folder': array([57868], dtype=int32),
'filelist': ['D:\\W2_DATA\\kbarber\\2025-02-27\\mk301\\assembled\\plane_01.tiff'],
'nframes': 57868,
'frames_per_file': array([57868]),
'meanImg': array([[293.30328, 232.32823, 168.23618, ..., 521.57465, 464.1154 ,
394.5214 ],
[284.06448, 226.78777, 163.6352 , ..., 501.11826, 446.7953 ,
381.61337],
[266.5393 , 214.82086, 156.06839, ..., 466.04904, 416.06656,
357.26947],
...,
[301.4731 , 261.12186, 224.88362, ..., 577.2157 , 512.18005,
418.6066 ],
[303.3301 , 262.12506, 224.97882, ..., 576.16766, 515.00006,
427.4448 ],
[301.86884, 258.6367 , 218.3702 , ..., 563.2787 , 504.22775,
419.83896]], dtype=float32),
'Ly': 448,
'Lx': 448,
'yrange': [7, 441],
'xrange': [8, 440],
'date_proc': datetime.datetime(2025, 2, 27, 14, 26, 26, 751811, tzinfo=datetime.timezone(datetime.timedelta(days=-1, seconds=68400), 'Eastern Standard Time')),
'refImg': array([[ 62, 20, 10, ..., 531, 480, 259],
[ 54, 26, 7, ..., 451, 387, 221],
[ 85, 31, 6, ..., 431, 393, 241],
...,
[135, 62, 57, ..., 574, 524, 251],
[153, 63, 47, ..., 565, 507, 304],
[ 82, 41, 39, ..., 484, 434, 254]], dtype=int16),
'rmin': np.int16(12),
'rmax': np.int16(2860),
'yoff': array([-1, -1, -2, ..., -4, -4, -4], dtype=int32),
'xoff': array([ 0, -1, -1, ..., -4, -5, -5], dtype=int32),
'corrXY': array([0.02158369, 0.018543 , 0.01747612, ..., 0.01333473, 0.01218268,
0.0138828 ], dtype=float32),
'yoff1': array([[-0.1 , 0.1 , 0.3 , ..., 0. ,
-0.4 , -0.4 ],
[ 0. , 0.3 , 0.1 , ..., -0.7 ,
-1. , -1.4 ],
[-0.5 , -0.1 , 0.39999998, ..., -0.4 ,
-0.3 , -0.8 ],
...,
[-1.3 , -0.1 , -0.3 , ..., 0.8 ,
0.4 , 0.2 ],
[ 0.1 , -0.6 , -1. , ..., 2.5 ,
0.7 , 0.5 ],
[-0.3 , 0.7 , -0.2 , ..., 0.3 ,
0.7 , 0.5 ]], dtype=float32),
'xoff1': array([[-0.7, -0.6, -0.4, ..., -0.2, -0.4, -0.3],
[-0.4, 0.2, 0.9, ..., 0.1, 0.3, 0.7],
[-0.9, -1.2, -0.3, ..., -0.3, 0. , 0. ],
...,
[-1.5, -0.7, -0.8, ..., -0.5, 0.3, 0.4],
[ 0.1, -0.6, -0.5, ..., 0.1, 0.9, 1.2],
[-0.7, -0.5, -0.7, ..., -0.3, 0.6, 0.7]], dtype=float32),
'corrXY1': array([[0.01925137, 0.01940679, 0.01934982, ..., 0.01214676, 0.02207752,
0.01830108],
[0.01296142, 0.01474211, 0.01633533, ..., 0.01063509, 0.02069657,
0.014935 ],
[0.01563133, 0.01439206, 0.01608265, ..., 0.01040511, 0.02162057,
0.01748876],
...,
[0.0122851 , 0.01224983, 0.01089673, ..., 0.0104221 , 0.02398453,
0.02038603],
[0.01228525, 0.01254859, 0.0088719 , ..., 0.00895512, 0.02062548,
0.02032768],
[0.01201922, 0.01257571, 0.01276607, ..., 0.01179439, 0.02362394,
0.02025481]], dtype=float32),
'badframes': array([False, False, False, ..., False, False, False]),
'spatscale_pix': array([6]),
'meanImgE': array([[1., 1., 1., ..., 1., 1., 1.],
[1., 1., 1., ..., 1., 1., 1.],
[1., 1., 1., ..., 1., 1., 1.],
...,
[1., 1., 1., ..., 1., 1., 1.],
[1., 1., 1., ..., 1., 1., 1.],
[1., 1., 1., ..., 1., 1., 1.]], dtype=float32),
'tPC': array([[ 3.76147106e-02, -2.67174281e-02, 8.22716802e-02, ...,
-2.24205386e-02, 3.34519483e-02, 4.16396651e-03],
[ 3.28002535e-02, 1.18480464e-02, 1.10959694e-01, ...,
-8.69029853e-03, 7.48462230e-03, 1.68417562e-02],
[ 1.63297374e-02, 2.94245910e-02, 4.69198748e-02, ...,
-8.59191827e-03, 3.71028646e-03, -6.98513864e-03],
...,
[-2.81411707e-02, 4.38331394e-03, 1.66360959e-02, ...,
-1.17876763e-02, 1.84267499e-02, -8.43129121e-04],
[-2.66001578e-02, 2.46010404e-02, 3.82185169e-03, ...,
-5.33293420e-03, 1.46578357e-03, 4.28768992e-03],
[-2.58081928e-02, 2.10867506e-02, -1.27776908e-02, ...,
-5.53919189e-03, 1.05655589e-03, -9.19369049e-05]], dtype=float32),
'regPC': array([[[[ 13.526667 , 13.743333 , 8.82 , ..., 374.53333 ,
397.67 , 389.74667 ],
[ 9.1866665, 7.4 , 4.1233335, ..., 407.82666 ,
413.99667 , 412.65668 ],
[ 6.443333 , 7.63 , 8.156667 , ..., 413.60666 ,
426.74 , 411.58 ],
...,
[ 91.543335 , 93.53 , 108.456665 , ..., 502.34668 ,
512.8233 , 519.4733 ],
[ 98.083336 , 101.58667 , 109.14667 , ..., 518.88336 ,
510.21667 , 520.8233 ],
[ 92.083336 , 95.73333 , 100.776665 , ..., 513.06 ,
537.73334 , 544.78 ]],
[[ 8.333333 , 5.5466666, 7.84 , ..., 468.00665 ,
456.39334 , 491.16 ],
[ 9.163333 , 6.863333 , 10.053333 , ..., 505.45334 ,
508.48334 , 502.25665 ],
[ 12.326667 , 10.823334 , 11.483334 , ..., 531.93665 ,
541.51 , 509.52335 ],
...,
[100.276665 , 104.46 , 107.89 , ..., 579.37 ,
604.94336 , 609.2267 ],
[ 88.94666 , 103.246666 , 113.206665 , ..., 617.97 ,
613.49664 , 598.55664 ],
[ 91.32333 , 97.263336 , 102.89667 , ..., 628.2167 ,
609.39 , 589.24 ]],
[[ 7.0533333, 9.296667 , 7.1433334, ..., 434.07666 ,
415.12668 , 415.12332 ],
[ 11.54 , 12.503333 , 7.306667 , ..., 461.58667 ,
437.19333 , 439.36334 ],
[ 10.3 , 7.02 , 10.273334 , ..., 473.96335 ,
471.77335 , 469.8 ],
...,
[102.46667 , 106.166664 , 121.05334 , ..., 546.9867 ,
515.88 , 535.47 ],
[ 91.456665 , 99.23333 , 100.34333 , ..., 537.61664 ,
526.1633 , 522.86 ],
[ 86.67667 , 105.9 , 102.96333 , ..., 557.87665 ,
531.9267 , 540.55 ]],
...,
[[ 6.036667 , 5.8966665, 10.293333 , ..., 471.85333 ,
445.10333 , 445.05334 ],
[ 7.96 , 7.31 , 10.023334 , ..., 476.55334 ,
466.17334 , 460.61334 ],
[ 16.743334 , 9.623333 , 9.4 , ..., 491.53333 ,
500.56 , 481.07666 ],
...,
[109.973335 , 103.253334 , 110.07 , ..., 548.14 ,
570.4 , 547.89 ],
[ 96.36 , 101.42333 , 114.07 , ..., 568.86334 ,
582.29333 , 562.96 ],
[101.17333 , 114.42 , 107.44666 , ..., 603.2233 ,
591.5333 , 597.33 ]],
[[ 6.38 , 8.286667 , 12.026667 , ..., 464.14667 ,
472.16666 , 448.75665 ],
[ 9.106667 , 9.28 , 11.116667 , ..., 458.64667 ,
466.9 , 457.61334 ],
[ 11.706667 , 9.413333 , 7.84 , ..., 485.55667 ,
483.34332 , 469.22333 ],
...,
[117.76 , 105.10333 , 120.50667 , ..., 567.4767 ,
533.5 , 556.71 ],
[104.61 , 96.76 , 117.23333 , ..., 561.13336 ,
575.7267 , 549.3 ],
[101.596664 , 100.20333 , 113.07333 , ..., 586.7733 ,
581.29333 , 580.43665 ]],
[[ 8.856667 , 8.816667 , 6.8133335, ..., 447.68 ,
447.02667 , 444.89 ],
[ 8.953333 , 7.82 , 8.266666 , ..., 466.47333 ,
478.85 , 441.77 ],
[ 9.926666 , 7.5666666, 5.1666665, ..., 491.48 ,
504.29 , 456.27335 ],
...,
[101.88333 , 117.55334 , 115.97 , ..., 561.47 ,
557.2733 , 575.55664 ],
[ 96.346664 , 108.27 , 103.263336 , ..., 569.1767 ,
553.23 , 550.21 ],
[100.89667 , 110.89 , 111.776665 , ..., 567.9133 ,
561.93 , 588.19336 ]]],
[[[ 10.54 , 7.8 , 7.286667 , ..., 536.41 ,
522.26666 , 497.58667 ],
[ 12.966666 , 11.163333 , 15.286667 , ..., 538.52 ,
551.3233 , 525.69666 ],
[ 4.5933332, 5.43 , 6.3533335, ..., 549.87335 ,
544.88 , 522.7133 ],
...,
[115.29 , 126.363335 , 146.10333 , ..., 633.66 ,
633.12 , 627.37335 ],
[108.71 , 114.40667 , 127.90667 , ..., 610.0267 ,
631.7867 , 590.59 ],
[119.79667 , 119.93667 , 116.51 , ..., 607.01666 ,
611.36 , 576.56 ]],
[[ 9.283334 , 11.823334 , 10.876667 , ..., 417.56332 ,
413.79666 , 425.01 ],
[ 12.836667 , 12.766666 , 10.47 , ..., 439.86334 ,
437.63666 , 430.01 ],
[ 12.7 , 10.503333 , 5.326667 , ..., 449.19 ,
444.98334 , 432.83 ],
...,
[117.03333 , 122.583336 , 125.513336 , ..., 535.6633 ,
531.7167 , 510.87668 ],
[100.42333 , 103.886665 , 113.35667 , ..., 552.24 ,
548.44666 , 513.93335 ],
[103.87334 , 102.50667 , 113.47667 , ..., 529.04 ,
556.43335 , 549.0867 ]],
[[ 12.6866665, 10.496667 , 9.72 , ..., 476.12 ,
470.01666 , 464.76666 ],
[ 14.683333 , 12.633333 , 13.8133335, ..., 474.55 ,
490.97 , 463.45667 ],
[ 10.603333 , 7.903333 , 9.906667 , ..., 495.00665 ,
513.9533 , 484.05667 ],
...,
[109.513336 , 119.82333 , 129.33333 , ..., 596.20667 ,
576.6433 , 579.61664 ],
[106.62 , 115.85333 , 121.67333 , ..., 607.0367 ,
601.1067 , 566.3 ],
[116.456665 , 104.916664 , 109.56 , ..., 618.79333 ,
588.63336 , 601.33 ]],
...,
[[ 7.9366665, 6.113333 , 5.363333 , ..., 449.06 ,
485.53333 , 440.84668 ],
[ 11.893333 , 8.456667 , 7.7466664, ..., 471.47 ,
492.53 , 468.77 ],
[ 8.643333 , 10.16 , 6.8166666, ..., 516.99 ,
492.01 , 485.89 ],
...,
[111.32667 , 108.776665 , 126.596664 , ..., 570.2 ,
550.25336 , 569.32 ],
[105.24333 , 106.63333 , 108.84 , ..., 602.45667 ,
588.2367 , 548.8567 ],
[110.15 , 105.15 , 107.77 , ..., 606.68 ,
615.8233 , 587.0967 ]],
[[ 10.94 , 14.286667 , 12.633333 , ..., 470.62 ,
456.4 , 460.83667 ],
[ 9.723333 , 8.01 , 9.516666 , ..., 465.73334 ,
483.52 , 506.40332 ],
[ 8.14 , 10.193334 , 5.3333335, ..., 505.86667 ,
507. , 497.58334 ],
...,
[111.24333 , 99.97 , 114.69334 , ..., 582.38 ,
605.99335 , 570.32 ],
[103.86 , 96.06 , 115.21667 , ..., 571.03 ,
551.13666 , 589.62665 ],
[100.26667 , 104.29 , 106.19334 , ..., 605.32 ,
577.15 , 594.42334 ]],
[[ 5.35 , 5.17 , 6.5666666, ..., 443.24335 ,
441.32 , 439.37668 ],
[ 10.22 , 3.9833333, 10.3 , ..., 472.13666 ,
467.25333 , 488.27667 ],
[ 8.096666 , 7.7166667, 8.323334 , ..., 477.92667 ,
493.98 , 494.25333 ],
...,
[114.40667 , 111.11 , 110.816666 , ..., 577.52 ,
576.89 , 576.8367 ],
[107.95333 , 101.996666 , 107.12666 , ..., 568.7733 ,
564.49 , 543.95 ],
[ 99.87666 , 113.46333 , 109.92 , ..., 587.63666 ,
578.7233 , 583.08 ]]]], dtype=float32),
'regDX': array([[0. , 0.26460087, 0.70710677],
[0. , 0.04855197, 0.22360681],
[0. , 0.07489996, 0.22360681],
[0. , 0.11297831, 0.30000001],
[0. , 0.1229571 , 0.30000001],
[0. , 0.06021283, 0.36055514],
[0. , 0.09202497, 0.40000001],
[0. , 0.0580359 , 0.22360681],
[0. , 0.05019741, 0.28284273],
[0. , 0.06751981, 0.22360681],
[0. , 0.0518246 , 0.2 ],
[0. , 0.0636097 , 0.2 ],
[0. , 0.05248034, 0.22360681],
[0. , 0.05410753, 0.22360681],
[0. , 0.04644819, 0.22360681],
[0. , 0.0562662 , 0.36055514],
[0. , 0.05198549, 0.22360681],
[0. , 0.05410752, 0.22360681],
[0. , 0.05640871, 0.22360681],
[0. , 0.05640871, 0.22360681],
[0. , 0.04740138, 0.22360681],
[0. , 0.0390863 , 0.28284273],
[0. , 0.06377061, 0.22360681],
[0. , 0.05410753, 0.22360681],
[0. , 0.05248034, 0.22360681],
[0. , 0.06883131, 0.22360681],
[0. , 0.06456289, 0.2 ],
[0. , 0.04299641, 0.22360681],
[0. , 0.04855197, 0.22360681],
[0. , 0.05410753, 0.22360681]]),
'Lyc': 434,
'Lxc': 432,
'max_proj': array([[ 46.620384, 35.208847, 51.66577 , ..., 208.57733 , 187.53116 ,
149.41336 ],
[ 45.53692 , 41.455 , 38.901154, ..., 174.70178 , 163.37439 ,
176.69644 ],
[ 46.35115 , 42.589615, 46.69154 , ..., 219.80383 , 227.54004 ,
204.86887 ],
...,
[ 79.58886 , 114.85497 , 91.0754 , ..., 205.17432 , 194.49811 ,
192.78265 ],
[ 74.388084, 79.02847 , 87.17464 , ..., 222.57721 , 216.2738 ,
175.62433 ],
[ 82.01308 , 84.74691 , 89.561134, ..., 199.10529 , 214.18573 ,
205.28998 ]], dtype=float32),
'Vmax': array([134.35905457, 123.99681854, 119.96086121, ..., 0. ,
0. , 0. ]),
'ihop': array([1., 1., 1., ..., 0., 0., 0.]),
'Vsplit': array([0.9956013 , 1.00115836, 1.03165114, ..., 0. , 0. ,
0. ]),
'Vcorr': array([[2.41070247, 3.0349021 , 2.63656162, ..., 2.86526976, 3.08645248,
2.88534212],
[3.61344194, 3.94789934, 4.09441757, ..., 3.02664685, 3.26891899,
2.88534212],
[3.45880699, 3.82495308, 3.47255468, ..., 3.23110683, 3.03284302,
3.03284302],
...,
[3.07509966, 3.08906794, 3.47316933, ..., 3.97715587, 4.16869164,
3.97497858],
[3.05471516, 3.62808466, 3.27154589, ..., 3.41256595, 3.33516124,
3.33516124],
[2.91497087, 3.34653044, 2.86425415, ..., 3.09711958, 2.9695015 ,
2.9695015 ]]),
'Vmap': array([array([[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.],
...,
[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.]], dtype=float32),
array([[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
[0. , 0. , 0. , ..., 0. , 5.9854507,
0. ],
...,
[0. , 0. , 0. , ..., 5.1922097, 0. ,
0. ],
[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
[0. , 0. , 0. , ..., 0. , 0. ,
0. ]], dtype=float32) ,
array([[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
...,
[0. , 0. , 0. , ..., 0. , 0. ,
0. ],
[0. , 0. , 0. , ..., 5.1405945, 5.261818 ,
0. ],
[0. , 0. , 0. , ..., 0. , 0. ,
0. ]], dtype=float32) ,
array([[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.],
...,
[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.],
[0., 0., 0., ..., 0., 0., 0.]], dtype=float32),
array([[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 5.8577175, 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 9.073908 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 10.049647 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 5.685844 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 7.262233 ,
5.1286793, 5.592461 , 7.8516684, 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 5.577756 , 7.207178 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 6.505272 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 6.261672 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 6.987685 , 0. , 0. ,
0. , 0. , 0. , 6.098998 , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 9.743567 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 9.648951 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
5.1595583, 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 11.347019 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
5.2799683, 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 12.564106 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 10.0486765, 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ],
[ 0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 5.155319 , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. , 0. , 0. , 0. ,
0. , 0. ]], dtype=float32) ],
dtype=object),
'timing': {'registration': 641.9894878864288,
'registration_metrics': 15.547569990158081,
'detection': 46.35976839065552,
'extraction': 43.62881112098694,
'classification': 0.03134584426879883,
'deconvolution': 0.7660236358642578,
'total_plane_runtime': 748.6714560985565}}
volume_stats_file = lbm_suite2p_python.volume.get_volume_stats(ops_files)
volume_stats_file # runs stats and gives back the filename
'D:\\W2_DATA\\kbarber\\2025-02-17\\mk303\\results\\volume_stats.npy'
# plane, acc, rej, means, stds, ops-file
print(np.load(volume_stats_file, allow_pickle=True))
[( 1, 601, 601, 1504.95532227, 635.71911621, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_01_demo\\plane0\\ops.npy')
( 2, 709, 709, 1729.17712402, 759.26446533, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_02_demo\\plane0\\ops.npy')
( 3, 840, 840, 1682.04052734, 771.42297363, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_03_demo\\plane0\\ops.npy')
( 4, 937, 937, 1581.18676758, 803.08453369, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_04_demo\\plane0\\ops.npy')
( 5, 1163, 1163, 1454.5435791 , 746.58349609, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_05_demo\\plane0\\ops.npy')
( 6, 1306, 1306, 1436.0411377 , 701.63793945, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_06_demo\\plane0\\ops.npy')
( 7, 537, 537, 55.10420609, 25.497509 , 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_07_demo\\plane0\\ops.npy')
( 8, 1572, 1572, 1194.73181152, 573.11859131, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_08_demo\\plane0\\ops.npy')
( 9, 1697, 1697, 1068.63830566, 499.10424805, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_09_demo\\plane0\\ops.npy')
(10, 1678, 1678, 1031.76330566, 505.93670654, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_10_demo\\plane0\\ops.npy')
(11, 1598, 1598, 913.86999512, 463.93460083, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_11_demo\\plane0\\ops.npy')
(12, 1128, 1128, 723.86236572, 390.0612793 , 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_12_demo\\plane0\\ops.npy')
(13, 730, 730, 606.41137695, 329.38095093, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_13_demo\\plane0\\ops.npy')
(14, 398, 398, 480.65631104, 255.62088013, 'D:\\W2_DATA\\kbarber\\2025-02-10\\mk303\\results\\plane_14_demo\\plane0\\ops.npy')]
stat_files = mbo.get_files(save_path.parent, 'stat.npy', max_depth=5)
stat_files[:3]
['D:\\W2_DATA\\kbarber\\2025-02-17\\mk303\\results\\plane_01\\plane0\\stat.npy',
'D:\\W2_DATA\\kbarber\\2025-02-17\\mk303\\results\\plane_02\\plane0\\stat.npy',
'D:\\W2_DATA\\kbarber\\2025-02-17\\mk303\\results\\plane_03\\plane0\\stat.npy']
3.2. Compare multiple z-planes#
plane_6 = tifffile.memmap(input_files[5])
plane_7 = tifffile.memmap(input_files[6])
iw = fpl.ImageWidget(data=[plane_6, plane_7], graphic_kwargs={"vmin": -300, "vmax": 12000})
iw.show()
Draw error
Traceback (most recent call last):
File "C:\Users\RBO\miniforge3\envs\lsp\lib\site-packages\rendercanvas\_coreutils.py", line 41, in log_exception
yield
File "C:\Users\RBO\miniforge3\envs\lsp\lib\site-packages\rendercanvas\base.py", line 409, in _draw_frame_and_present
self._draw_frame()
File "C:\Users\RBO\miniforge3\envs\lsp\lib\site-packages\fastplotlib\layouts\_imgui_figure.py", line 111, in _render
self.imgui_renderer.render()
File "C:\Users\RBO\miniforge3\envs\lsp\lib\site-packages\wgpu\utils\imgui\imgui_renderer.py", line 161, in render
render_pass.end()
File "C:\Users\RBO\miniforge3\envs\lsp\lib\site-packages\wgpu\backends\wgpu_native\_api.py", line 3289, in end
libf.wgpuRenderPassEncoderEnd(self._internal)
File "C:\Users\RBO\miniforge3\envs\lsp\lib\site-packages\wgpu\backends\wgpu_native\_helpers.py", line 394, in proxy_func
raise wgpu_error # the frame above is more interesting ↑↑
wgpu._classes.GPUValidationError: Validation Error
Caused by:
In wgpuRenderPassEncoderEnd
In a set_viewport command
Viewport has invalid rect Rect { x: 0.0, y: 0.0, w: 500.0, h: 300.0 }; origin and/or size is less than or equal to 0, and/or is not contained in the render target (400, 240, 1)